You've seen how to place numbers into an array. But you can also place strings
of text. In the next section, we're going to be studying the subject of Strings
more closely. We're going to develop a hangman game that makes use of arrays
and text. So a reasonable understanding of how to place text into an array will
make the going easier!
Add a new button to your form, and set the Text property to String Arrays. Then double click your button to get at the code.
To place text into an array, you set up your array as you normally would, except you use the keyword string instead of int. Like this:
Add the code above to your button.
To place values in the array, it's just normal variable assignment, with a pair of square brackets after the variable name:
Notice that for the array, you don't need the square brackets anymore. The
neat thing about foreach loops is that you don't need to use index numbers,
like you do with ordinary for loops.
Run your programme and click the button. The list box on your form should then look like ours:
As we said, you'll work with string arrays in the next section, so we'll leave
them for now. The final part of this section deals with objects closely associated
with arrays - collections.
Add a new button to your form, and set the Text property to String Arrays. Then double click your button to get at the code.
To place text into an array, you set up your array as you normally would, except you use the keyword string instead of int. Like this:
string[] arrayStrings;
arrayStrings = new string[5];
So the above code would set up an array that is going to hold strings of text.
There will be five positions in the array.arrayStrings = new string[5];
Add the code above to your button.
To place values in the array, it's just normal variable assignment, with a pair of square brackets after the variable name:
arrayStrings[0] = "This";
arrayStrings[1] = "is";
arrayStrings[2] = "a";
arrayStrings[3] = "string";
arrayStrings[4] = "array";
Add the above to your code, and then we'll discuss ForEach Loops.arrayStrings[1] = "is";
arrayStrings[2] = "a";
arrayStrings[3] = "string";
arrayStrings[4] = "array";
The ForEach Loop
To access the values in each position of your array, you could use a for loop, as you have been doing. Like this:
for (int i = 0; i != (arrayStrings.Length); i++)
{
{
listBox1.Items.Add( arrayStrings[i] );
}
But there's another type of loop that you haven't met yet called a foreach
loop. This comes in handy when you're trying to access items in a collection,
which you'll see how to do shortly. But add this to your code, instead of a
for loop:
foreach ( string arrayElement in arrayStrings )
{
{
listBox1.Items.Add( arrayElement );
}
Notice where all the keywords are in the loop code above, the ones in blue.
You start with the foreach keyword, followed by a pair of round brackets.
In between the round brackets, we have this:
string arrayElement in arrayStrings
This is really two parts in one. In the first part, string arrayElement,
you set up a new variable. The new variable will hold the elements (array values)
from each position in your array. The second part, in arrayStrings, is
where you tell C# the name of the array or collection you want to access. C#
will then loop round all the positions in your array, and place the value at
that position into your new variable (arrayElement, for us). You can
then do something with the value in the new variable. All we are doing in our
loop is to display the value in a list box. Here's a colour-coded image that
may help you to understand what's going on:
Run your programme and click the button. The list box on your form should then look like ours:
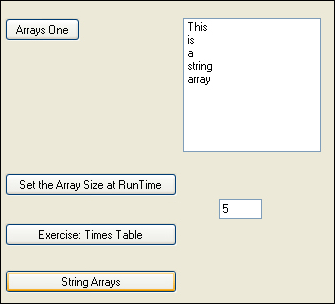
0 comments:
Post a Comment